Start with full picture
c++ specials
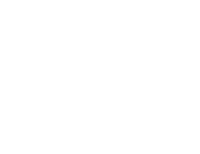
“There are only two kinds of languages: the ones people complain about and the ones nobody uses.”
– Bjarne Stroustrup
Lessons
Lessons
- Stream Input/Output
- Stream Input/Output - Tips and QA
- Exception Handling
- Exception Handling - Tips and QA
- File Processing
- File Processing - Tips and QA
- Class string and String Stream Processing
- Class string and String Stream Processing - Tips and QA
- Bits, Characters, C-Strings and structs
- Bits, Characters, C-Strings and structs - Tips and QA
- Other Topics
- Other Topics - Tips and QA
OBJECTIVES
In this chapter you will learn:
- To use C++ object-oriented stream input/output
- To format input and output
- The stream-I/O class hierarchy
- To use stream manipulators
To control justification and padding.
- To determine the success or failure of input/output operations
- To tie output streams to input streams
- What exceptions are and when to use them
- To use TRy , catch and throw to detect, handle and indicate exceptions, respectively
- To process uncaught and unexpected exceptions
- To declare new exception classes
- How stack unwinding enables exceptions not caught in one scope to be caught in another scope
- To handle new failures
- To use auto_ptr to prevent memory leaks
- To understand the standard exception hierarchy
- To create, read, write and update files
- Sequential file processing
- Random-access file processing
- To use high-performance unformatted I/O operations
- The differences between formatted-data and raw-data file processing
- To build a transaction-processing program using random-access file processing
- To use class string from the C++ Standard Library to treat strings as full-fledged objects
- To assign, concatenate, compare, search and swap strings
- To determine string characteristics
- To find, replace and insert characters in a string
- To convert strings to C-style strings and vice versa
- To use string iterators
- To perform input from and output to strings in memory
- To create and use structs
- To pass structs to functions by value and by reference
- To use ty pedef to create aliases for previously defined data types and structs
- To manipulate data with the bitwise operators and to create bit fields for storing data compactly
- To use the functions of the character-handling library
- To use the string-conversion functions of the general-utilities library <cstdlib>
- To use the string-processing functions of the string-handling library <cstring>
- To use to const_cast to temporarily treat a const object as a non-const object
- To use namespaces
- To use operator keywords
- To use mutable members in const objects
- To use class-member pointer operators .* and ->*
- To use multiple inheritance
- The role of v irtual base classes in multiple inheritance
HOMEWORKS
Lessons 1 and 2 | D 15.6 | D 15.7 | D 15.8 | D 15.9 | D 15.10 |
---|---|---|---|---|
D 15.11 | D 15.12 | D 15.13 | D 15.14 | D 15.15 |
D 15.16 | D 15.17 | D 15.18 | ||
Lessons 3 and 4 | D 16.18 | D 16.19 | D 16.20 | D 16.21 | D 16.22 |
---|---|---|---|---|
D 16.23 | D 16.24 | D 16.25 | D 16.26 | D 16.27 |
D 16.28 | D 16.29 | D 16.30 | D 16.31 | D 16.32 |
D 16.33 | D 16.34 | D 16.35 | D 16.36 |
Lessons 5 and 6 | D 17.5 | D 17.6 | D 17.7 | D 17.8 | D 17.9 |
---|---|---|---|---|
D 17.10 | D 17.11 | D 17.12 | D 17.13 | D 17.14 |
Lessons 7 and 8 | D 18.4 | D 18.5 | D 18.6 | D 18.7 | D 18.8 |
---|---|---|---|---|
D 18.9 | D 18.10 | D 18.11 | D 18.12 | D 18.13 |
D 18.14 | D 18.15 | D 18.16 | D 18.17 | D 18.18 |
D 18.19 | D 18.20 | D 18.21 | D 18.22 | D 18.23 |
D 18.24 | D 18.25 | D 18.26 | D 18.27 | D 18.28 |
Lessons 9 and 10 | D 22.6 | D 22.7 | D 22.8 | D 22.9 | D 22.10 |
---|---|---|---|---|
D 22.11 | D 22.12 | D 22.13 | D 22.14 | D 22.15 |
D 22.16 | D 22.17 | D 22.18 | D 22.19 | D 22.20 |
D 22.21 | D 22.22 | D 22.23 | D 22.24 | D 22.25 |
D 22.26 | D 22.27 | D 22.28 | D 22.29 | D 22.30 |
D 22.31 | D 22.32 | D 22.33 | D 22.34 | D 22.35 |
Lessons 11 and 12 | D 24.3 | D 24.4 | D 24.5 | D 24.6 | D 24.7 |
---|---|---|---|---|
D 24.8 | D 24.9 | D 24.10 |
- These are the lessons and home works that are mandatory for students who are going to enroll in "C++ Basics" course.